We'll build here a SPA app which shows an item list, and allows you to append more items to it.
You can learn this step-by-step tutorial as a standalone, or you can see the previous lessons of the series. This is the Lesson #13 in the "AngularJS: From 0 To 100" Series specially written for Beginners. This lessons begin at the Lesson #1 .
Also, this tutorial contains two parts:
1) creating an SPA AngularJS app
2) adding the Twitter Bootstrap to an existing AngularJS Application
Therefore, if you already have an SPA app, you can directly jump to the Step 2.
<<<< PREVIOUS LESSON NEXT LESSON >>>>
This is the AngularJS App that we'll develop in 20 minutes in this Tutorial :
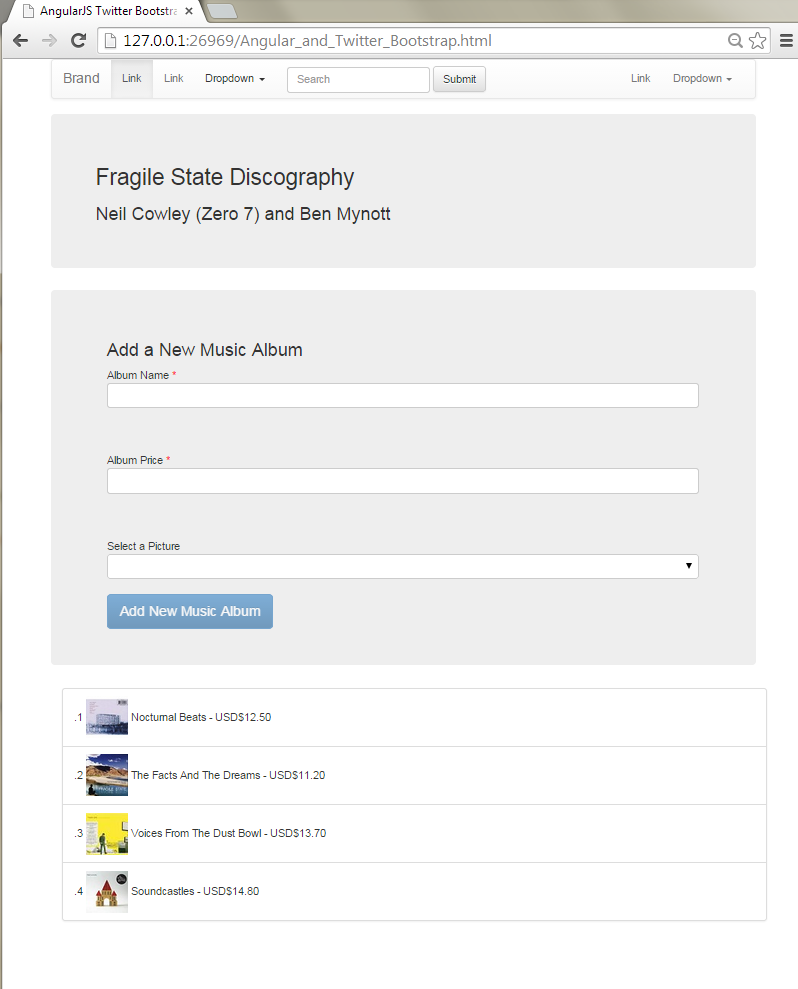
Add CSS3 Style to an AngularJS Application with Twitter Bootstrap
Step #1 - Creating an SPA (Single Page Application) with AngularJS:
First, let's add the references to the AngularJS javascript framework using CDN, and defining the HTML5 file as an data-ng-app Angular App:
(Get the source code):
<!doctype html>
<html data-ng-app="MusicApp">
<head>
<title>
AngularJS Twitter Bootstrap
</title>
<link href="Style.css" rel="stylesheet" type="text/css" />
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.7/angular.min.js"></script>
<script src="Controllers.js" type="text/javascript"></script>
</head>
Here we're using the "Brackets" open source web Editor , which supports AngularJS Apps. You can read more about it in this post.
Our first step is coding a list of items using the data-ng-repeat AngularJS Directive:
We explained in detail this kind of AngularJS collections in this tutorial . Take a look if you have the time.
(Copy):
<body data-ng-controller="MusicController">
<div>
<div>
<h2>Fragile State Discography</h2>
<h3>Neil Cowley (Zero 7) and Ben Mynott</h3>
</div>
<div>
<ul>
<li data-ng-repeat="album in albums">
.{{$index + 1}}
<img src="{{album.img}}" alt="{{album.name}}" >
<span >{{album.name}} - {{album.price | currency:"USD$" | uppercase}}</span>
</li>
</ul><br />
</div>
</div>
</body>
</html>
The Controller part of this MVC (Model View Controller) AngularJS SPA App consists in this code that we add to the javascript .js file:
This code just initialize the item's list we're building.
(Copy):
var oMusicApp = angular.module('MusicApp', []);
oMusicApp.controller('MusicController', [ '$scope', function ($scope) {
var albums =
[
{id:111,img:"Nocturnal.jpg",name:"Nocturnal Beats",price:"12.50"},
{id:112,img:"Facts.jpg",name:"The Facts And The Dreams",price:"11.20"},
{id:113,img:"Voices.jpg",name:"Voices From The Dust Bowl",price:"13.70"},
{id:114,img:"Pretz.jpg",name:"Soundcastles",price:"14.80"}
]
$scope.albums = albums;
}]);
Run the App to see if everything's OK:
Next, we build the web form that allows us to append a new Music Album item to the list. We mimic here a real-world submit form with input checking. But, instead of sending the data form to a web server, we handle the submit event and immediately add the item to the list:
Take a close look: our form includes several HTML5 input elements, with the correspondent validation and error checking , using the Angular data-ng-disabled directive to disable the submit button in case that the input data do not comply with the legal input requirements.
We bind all the input data to a data-ng-model named "album", as its properties. For example, "album.name" , "album.price" , etc. At runtime, the Angular engine will take care of the entire "album" entity for us.
A deeper explanation of this validation features can be found in the following Tutorial on Forms Validation.
(Get the source code):
<div class="jumbotron">
<div class="container" >
<h3>Add a New Music Album</h3>
<form data-ng-submit="submitForm()" name="NewAlbumForm">
Album Name
<span data-ng-show="NewAlbumForm.Name.$error.required" class="errorMessage">*</span><br />
<input class="form-control" name="Name" data-ng-model="album.name"
required data-ng-minlength="3" /> <br />
<span class="alert alert-danger" data-ng-show="NewAlbumForm.Name.$error.minlength" class="errorMessage">Minimum length 3</span><br />
<br />Album Price
<span data-ng-show="NewAlbumForm.Price.$error.required" class="errorMessage">*</span><br />
<input class="form-control" type="number" name="Price" min="1"
data-ng-model="album.price" required><br /><br /><br />
Select a Picture<br />
<select class="form-control" data-ng-model="album.img"
data-ng-options="picture + '.jpg' as picture for picture in pictures" required>
</select><br />
<input class="btn btn-primary btn-lg" type="submit" value="Add New Music Album"
data-ng-disabled="NewAlbumForm.$invalid">
</form>
</div>
</div>
And at the Controller we add the following code to handle the submit event:
This code just loads the data for the select element, and appends a new "album" item to the list. Then, it cleans the "album" object, to allow adding more items . As you can see, Angular takes care of all the Album's properties.
(Copy):
$scope.pictures = ["Nocturnal","Facts","Voices","Pretz"];
$scope.submitForm = function() {
$scope.albums.push($scope.album);
$scope.album = {};
};
Step #2 - Adding the Twitter Bootstrap to an AngularJS SPA Application:
#First step: adding the Bootstrap references:
You can get the style using CDN (Content Delivery Network) just copying this links:
(Copy):
<link rel="stylesheet" href="http://netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap.min.css" />
<link rel="stylesheet" href="http://netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap-theme.min.css" />
Or you can download the latest versions from the Bootstrap web site:
#Second Step: adding the Bootstrap Navbar to the App:
Browse to the Bootstrap web site , and find the Navbar at the "Components" tab:
Copy-paste the HTML5 markup to a new HTML file in your directory:
Then, use the data-ng-include and src AngularJS directives to insert the Navbar inside the App:
<div data-ng-include src="'Navbar.html'"></div>
Notice the double quotes we used here: "' '" : it's very important!
#Third Step: add the Bootstrap classes to the <div>s elements :
Now we just decorate all divs and elements with the Bootstrap classes:
For instance, we use the "Jumbotron" component to give style to the title, as explained at the Bootstrap web site:
After you added the style, browse to your app and refresh it:
We can already see the Navbar and the Jumbotron.
Now we add the Bootstrap classes to the web form as follows:
We can choose another kind of button from the Bootstrap web site:
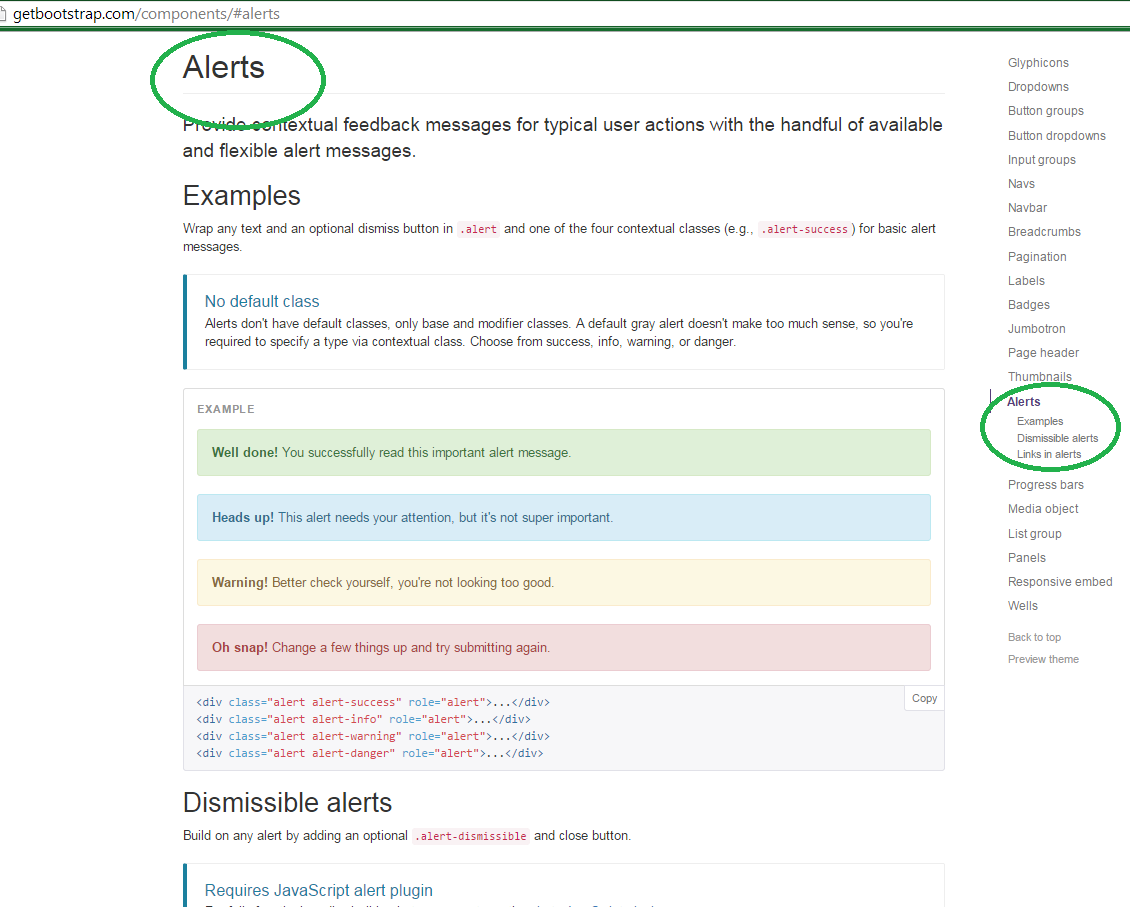
Refresh the SPA:
Now for the items list, add the Bootstrap Style classes as follows:
Refresh:
We have all the Bootstrap Style we need.
Now let's see how it works. Let's add a new "Fragile State" Music Album to the Collection:
Notice the Bootstrap Alert informing the user that the length requisites have not been provided.
Select a Price:
A new item has been appended to the list.
Finally, the only CSS3 style that we need is the following: (copy-paste the following CSS3 style to your style.css file) :
ul
{
list-style:none;
}
div.centered
{
text-align:center
}
.errorMessage
{
color:red;
}
}
Enjoy AngularJS.....
by Carmel Schvartzman
<<<< PREVIOUS LESSON NEXT LESSON >>>>
כתב: כרמל שוורצמן